Webhooks allows you to get updates delivered to your app server whenever we have events on our side. We will send a POST request over HTTPS, with serialized JSON data of the event, to the endpoint defined by you in the Developers > Webhooks section, in your merchant dashboard.
Retry policy
We will retry requests failed to connect with your server and transmit data. Using the exponential backoff algorithm, our service will send the same request repeatedly during a maximum period of 24h, distancing each attempt. This is important to help your app receive webhooks after a potential incident on your side, like a timeout error, downtime or any other possible problem.
Data integrity
To ensure data integrity, so you can verify the webhooks are sent by TelePay, we sign every request with an HTTP header, named Webhook-Signature
. The algorithm we use to sign every webhook request uses your API secret key (supposing you, and only you have it) and the request body (data).
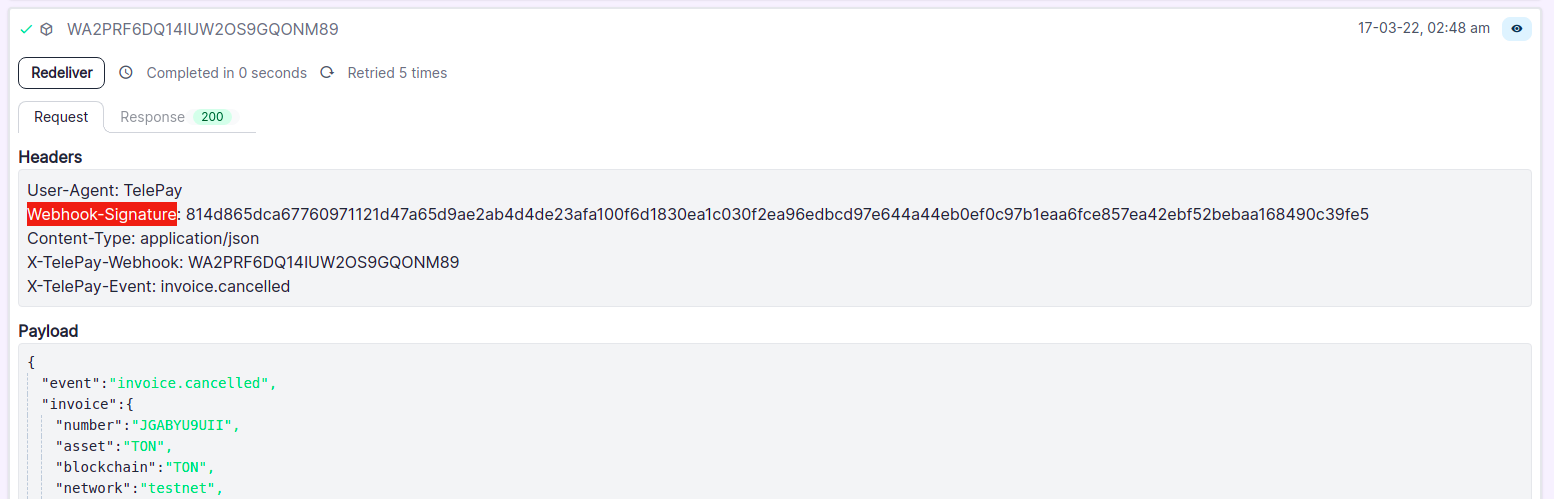
Webhook signature, in the HTTP request headers
Here's a a Python code sample on how we do it, and you can check it's us who's sending the webhook:
import hashlib
def get_signature(data, secret):
"""
Get the webhook signature using the request data and your secret API key
"""
hash_secret = hashlib.sha1(secret.encode()).hexdigest()
hash_data = hashlib.sha512(data.encode()).hexdigest()
signature = hashlib.sha512((hash_secret + hash_data).encode()).hexdigest()
return signature
And here's how you can check the webhook data integrity using Django:
import json
from django.http.response import HttpResponse
from django.views.decorators.csrf import csrf_exempt
from django.views.decorators.http import require_POST
@csrf_exempt
@require_POST
def listener(request):
body = json.decoder.JSONDecoder().decode(
request.body.decode()
)
data = str(body)
secret = 'YOUR SECRET' # The same secret you defined in dashboard
my_signature = get_signature(data, secret)
telepay_signature = request.headers['Webhook-Signature']
if my_signature == telepay_signature:
print('DATA IS CORRECT')
# Continue your webhook flow
return HttpResponse('Thanks TelePay')
Do NOT expose your webhook endpoint URL. We do sign our requests, but exposing your webhook URL could lead to potential security issues. Also, never share that secret URL with anyone.
Events currently supported
TelePay delivers events related to your invoices. These are our current webhook events, we'll be adding more in a near future:
all
invoice.created
invoice.completed
invoice.expired
invoice.cancelled
invoice.deleted
withdrawal.pending
withdrawal.auditing
withdrawal.approved
withdrawal.performing
withdrawal.confirming
withdrawal.completed
withdrawal.failed
Test webhooks
Also, if you need to test your webhooks integration, you can use the Webhook Simulator, available in your
Developers > Webhooks
section.